1-General introduction
The recent critical issue my customer experienced was that an application stopped sending emails for more than six months. And what was the reason behind this? It included using a solution that had been marked as deprecated and had been working all along-that is, until it did not work anymore. Of course, as we know, once something is marked as deprecated, it’s only a question of time before it gets retired completely.
The challenge was clear when they called me for help-to fix the problem in one day. Fortunately, my background as a full-stack.NET developer gave me an edge to understand their system in no time. After diving into their codebase, I found they were using some very outdated library for SMTP to handle their email functionality.
With time running out, I needed a modern and reliable solution that could be implemented quickly. Azure Communication Services immediately came to mind: robust, scalable, and fresh from general availability. Its email capability was just what I needed to replace the deprecated SMTP library.
I managed, within the same day, the integration of their application to Azure Communication Services, along with testing, making it live. And then there I went, making them have emails up by the end of that day. This made their team super happy as their updated modern solution was a good solution.
This was an experience, and it reminded me that familiarization with newer tools and technologies is priceless in solving various problems quickly and well, even when the pressure might be really high.
so what is Azure Communication Services ?
Azure Communication Services offers multichannel communication APIs for adding voice, video, chat, text messaging/SMS, email, and more to all your applications.
Azure Communication Services include REST APIs and client library SDKs, so you don’t need to be an expert in the underlying technologies to add communication into your apps. Azure Communication Services is available in multiple Azure geographies and Azure for government.
Azure Communication Services supports various communication formats:
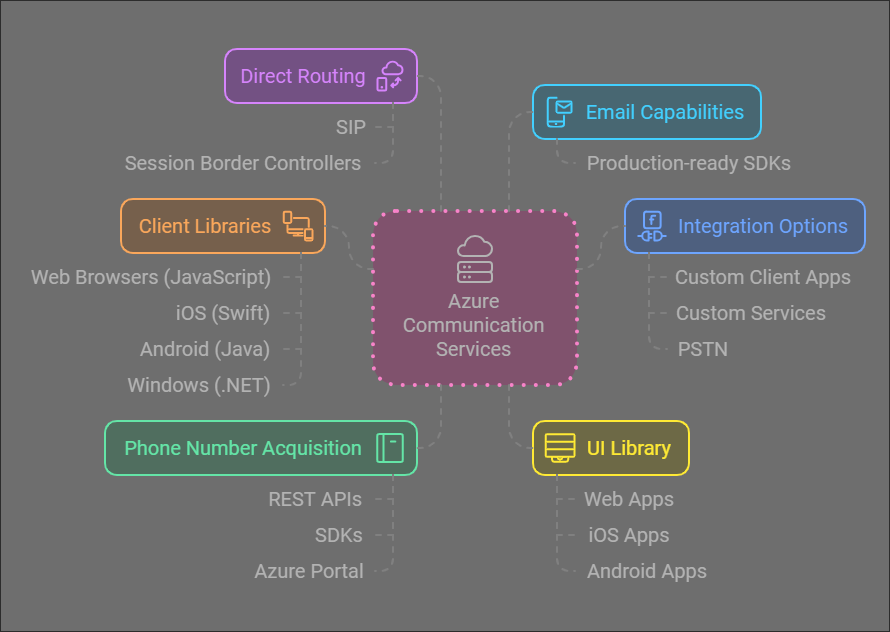
2-Setup Azure Communication Service with a custom domain name to send Email
First of all, you need to have a verified domain. In my use case, I already have a domain name in GoDaddy that I will be using for this demo.
So, let’s get started :
For SPF and DKIM configurations, certain TXT records have to be added to the DNS settings of your domain

- create email resource
3-Test Azure Communication Service from the portal



4- Use Azure Communication Service with C# (including SMTP)
now lets try the code provided from the portal :
using System; using System.Collections.Generic; using Azure; using Azure.Communication.Email; string connectionString = "endpoint=replace this with your endpoint"; var emailClient = new EmailClient(connectionString); var emailMessage = new EmailMessage( senderAddress: "DoNotReply@yourdomain.com", content: new EmailContent("Test Email from communication service") { PlainText = "Hello world via email from visual studio code.", Html = @" <html> <body> <h1>Hello world via email from visual studio code..</h1> </body> </html>" }, recipients: new EmailRecipients(new List<EmailAddress> { new EmailAddress("youremail@outlook.com") })); EmailSendOperation emailSendOperation = emailClient.Send( WaitUntil.Completed, emailMessage);
SMTP Sample
We will need to implement additional configurations such as custom role to optimize the SMTP sample and ensure seamless functionality.
using System.Net; using System.Net.Mail; string smtpAuthUsername = "<Azure Communication Services Resource name>|<Entra Application Id>|<Entra Application Tenant Id>"; string smtpAuthPassword = "Entra Application Client Secret"; string sender = "DoNotReply@yourdomain.com"; string recipient = "testemail@gmail.com"; string subject = "Welcome to Azure Communication Service Email SMTP"; string body = "This email message is sent from Azure Communication Service Email using SMTP."; string smtpHostUrl = "smtp.azurecomm.net"; var client = new SmtpClient(smtpHostUrl) { Port = 587, Credentials = new NetworkCredential(smtpAuthUsername, smtpAuthPassword), EnableSsl = true }; var message = new MailMessage(sender, recipient, subject, body); try { client.Send(message); Console.WriteLine("The email was successfully sent using Smtp."); } catch (Exception ex) { Console.WriteLine($"Smtp send failed with the exception: {ex.Message}."); }
6- Use Azure Communication Service with PowerShell
$Password = ConvertTo-SecureString -AsPlainText -Force -String 'Entra Application Client Secret' $Cred = New-Object -TypeName PSCredential -ArgumentList '<Azure Communication Services Resource name>|<Entra Application ID>|<Entra Tenant ID>', $Password Send-MailMessage -From 'DoNotReply@yourdomain.com' -To 'email to test' -Subject 'Test mail' -Body 'test' -SmtpServer 'smtp.azurecomm.net' -Port 587 -Credential $Cred -UseSsl
7- Sample : sending A news Letter to multi Emails with Attachment
Azure Communication Services also support sending emails to multiple recipients and attaching files. For more details, refer to this guide: Send email to multiple recipients
using System; using System.Collections.Generic; using System.IO; using System.Net.Mime; using System.Threading.Tasks; using Azure; using Azure.Communication.Email; namespace SendEmail { internal class Program { static async Task Main(string[] args) { // This code demonstrates how to fetch your connection string from an environment variable. string connectionString = "endpoint=............................................................"; EmailClient emailClient = new EmailClient(connectionString); // Create the email content var emailContent = new EmailContent("Newsletter from Achraf Ben Alaya") { PlainText = "This is the latest news from Achraf Ben Alaya's website.", Html = @" <html> <body> <h1>Latest News from Achraf Ben Alaya</h1> <p>Dear Subscriber,</p> <p>We are excited to share the latest updates from <a href='https://achrafbenalaya.com'>achrafbenalaya.com</a>.</p> <h2>New Features</h2> <ul> <li>Feature 1: Description of feature 1.</li> <li>Feature 2: Description of feature 2.</li> <li>Feature 3: Description of feature 3.</li> </ul> <h2>Upcoming Events</h2> <p>Stay tuned for our upcoming events:</p> <ul> <li>Event 1: Date and details of event 1.</li> <li>Event 2: Date and details of event 2.</li> </ul> <p>Thank you for being a valued subscriber.</p> <p>Best regards,<br/>Achraf Ben Alaya</p> </body> </html>" }; // Create the To list var toRecipients = new List<EmailAddress> { new EmailAddress("youremail@outlook.com"), new EmailAddress("ryle.kutler@finestudio.org"), }; // Create the CC list var ccRecipients = new List<EmailAddress> { new EmailAddress("ripejo9625@bawsny.com"), }; // Create the BCC list var bccRecipients = new List<EmailAddress> { new EmailAddress("wizorvlad@hulas.me"), }; EmailRecipients emailRecipients = new EmailRecipients(toRecipients, ccRecipients, bccRecipients); // Create the EmailMessage var emailMessage = new EmailMessage( senderAddress: "DoNotReply@ihelpyoutodo.com", emailRecipients, emailContent); // Add optional ReplyTo address which is where any replies to the email will go to. // emailMessage.ReplyTo.Add(new EmailAddress("<replytoemailalias@emaildomain.com>")); // Create the EmailAttachment var filePath = @""; byte[] bytes = File.ReadAllBytes(filePath); var contentBinaryData = new BinaryData(bytes); var emailAttachment = new EmailAttachment("micso.png", MediaTypeNames.Application.Pdf, contentBinaryData); emailMessage.Attachments.Add(emailAttachment); try { EmailSendOperation emailSendOperation = await emailClient.SendAsync(WaitUntil.Completed, emailMessage); Console.WriteLine($"Email Sent. Status = {emailSendOperation.Value.Status}"); // Get the OperationId so that it can be used for tracking the message for troubleshooting string operationId = emailSendOperation.Id; Console.WriteLine($"Email operation id = {operationId}"); } catch (RequestFailedException ex) { // OperationID is contained in the exception message and can be used for troubleshooting purposes Console.WriteLine($"Email send operation failed with error code: {ex.ErrorCode}, message: {ex.Message}"); } } } }
8 – Monitoring
Communications Services provides monitoring and analytics features via Azure Monitor Logs overview and Azure Monitor Metrics. Each Azure resource requires its own diagnostic setting :